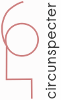
PHP class autoloader that follows the PSR-0 standard.
/**
* Register an autoload function.
*
* @param array $pairs Namespace : Path pairs.
*/
static function register_autoloader(array $pairs = array())
/**
* Autoload function.
*
* @param string $class_name The name of the class to load.
*/
static function autoload($class_name)
/**
* Load all files located at specific folders.
*
* @param array $folders Folders paths to load.
*/
static function folders(array $folders = array())
/**
* Load all given files.
*
* @param array $files Files paths to load.
*/
static function files(array $files = array())
/**
* Load given file.
*
* @param array $file File to load.
* @param mixed $default Return value if file not exists.
* @param array $args Associative array with variables to use in required file.
* @param array $return_vars Indexed array with variables to return from required file.
*/
static function file($file, $default = NULL, array $args = array(), array $return_vars = array())
Basic initialization:
require 'path/to/GrindNous/Loader.php';
GrindNous\Loader::register_autoloader();
Specify paths for different namespaces:
require 'path/to/GrindNous/Loader.php';
GrindNous\Loader::register_autoloader(array(
'MyNamespace' => '/path/to/namespace',
'MyNamespace\Models' => '/diferent/path/to/models',
'AnotherNamespace' => '/path/to/AnotherNamespace'
));
Class autoloading:
require 'GrindNous/Loader.php';
\GrindNous\Loader::register_autoloader(array(
'Classes' => 'Classes',
'ExtPack\Classes' => 'ExtPack/Classes',
'Classes\ExtPack2' => 'ExtPack2',
'Underscores' => 'Underscores'
));
echo 'Class: '.\Classes\One::$prop.' | Folder: Classes/One.php';
echo 'Class: '.\Classes\Utils\OneTool::$prop.' | Folder: Classes/Utils/OneTool.php';
echo 'Class: '.\ExtPack\Classes\ExtClass::$prop.' | Folder: ExtPack/Classes/ExtClass.php';
echo 'Class: '.\Classes\ExtPack2\AnotherExtClass::$prop.' | Folder: ExtPack2/AnotherExtClass.php';
echo 'Class: '.Underscores_Util_Name::$prop.' | Folder: Underscores/Util/Name.php';
In the above example $prop
would return the class name and namespace. This will print:
Class: Classes\One | Folder: Classes/One.php
Class: Classes\Utils\OneTool | Folder: Classes/Utils/OneTool.php
Class: ExtPack\Classes\ExtClass | Folder: ExtPack/Classes/ExtClass.php
Class: Classes\ExtPack2\AnotherExtClass | Folder: ExtPack2/AnotherExtClass.php
Class: Underscores_Util_Name | Folder: Underscores/Util/Name.php
Load file:
// incfolder/incfile.php
return array(
'key1' => 'value1',
'key2' => 'value2'
);
$res = \GrindNous\Loader::file('incfolder/incfile.php');
// $res
array(2) {
["key1"]=>
string(6) "value1"
["key2"]=>
string(6) "value2"
}
Load file sending variables:
// incfolder/incfile2.php
if( ! isset($var)) $var = FALSE;
return ($var === TRUE) ? 'OK' : 'FAIL' ;
$res = \GrindNous\Loader::file('incfolder/incfile2.php', NULL, array('var' => TRUE));
// $res
string(2) "OK"
Load file capturing variables:
// incfolder/incfile3.php
$loaded_file_var = 'Value from loaded file';
return array(
'key1' => 'value1',
'key2' => 'value2'
);
$res = \GrindNous\Loader::file('incfolder/incfile3.php', NULL, array('var' => TRUE), array('loaded_file_var'));
// $res
array(2) {
["__returned"]=>
array(2) {
["key1"]=>
string(6) "value1"
["key2"]=>
string(6) "value2"
}
["loaded_file_var"]=>
string(22) "Value from loaded file"
}